SwiftUIでコントロールセンターに音声ファイルのタイトル / アルバム名を表示し、再生 / 停止 / スキップ / シークを実行できるようにする方法を説明する。
Swift 5.7 / Xcode 14.0 / iOS 16.0
結論
タイトル / アルバム名を表示するには、MPNowPlayingInfoCenter を設定する。
再生 / 停止 / スキップ / シークを実行できるようにするには、MPRemoteCommand を設定する。
具体例
「音声を再生」ボタンをタップすると音声を再生するAppを作成する。
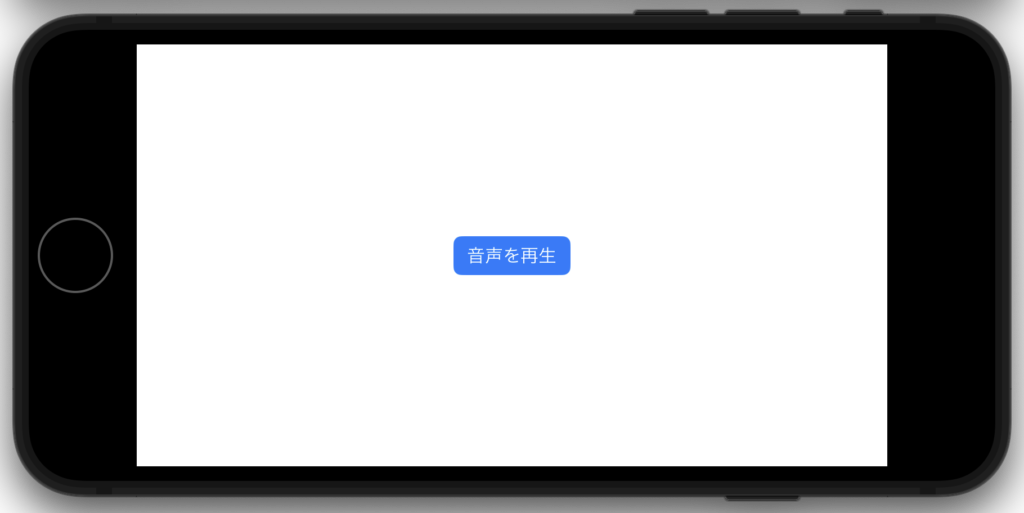
コントロールセンターを表示したところ。

- タイトルを設定する。
- アルバム名を設定する。
- playボタンの処理を設定する。
- pauseボタンの処理を設定する。
- 早送りボタンの処理を設定する。
- 巻き戻しボタンの処理を設定する。
- シークバーの横に時間を表示しシークバーを動かしたときの処理を設定する。
import SwiftUI
import MediaPlayer
struct ContentView: View {
var body: some View {
let プレイヤークラス = playerClass() // 👈 1 - 7
Button("音声を再生") {
プレイヤークラス.オーディオプレイヤー!.play()
}
.buttonStyle(.borderedProminent)
}
}
class playerClass {
var オーディオプレイヤー: AVAudioPlayer?
init() {
let ファイルURL = Bundle.main.url(forResource: "sample",
withExtension: "mp3")!
オーディオプレイヤー = try! AVAudioPlayer(contentsOf: ファイルURL)
setNowPlayingInfo() // 👈 1, 2, 7
setMPRemoteCommandCenter() // 👈 3 - 7
}
func setNowPlayingInfo() {
var nowPlayingInfo = [String : Any]()
nowPlayingInfo[MPMediaItemPropertyTitle] = "タイトル" // 👈 1
nowPlayingInfo[MPMediaItemPropertyAlbumTitle] = "アルバム名" // 👈 2
nowPlayingInfo[MPNowPlayingInfoPropertyElapsedPlaybackTime] = オーディオプレイヤー!.currentTime // 👈 7
nowPlayingInfo[MPMediaItemPropertyPlaybackDuration] = オーディオプレイヤー!.duration // 👈 7
nowPlayingInfo[MPNowPlayingInfoPropertyPlaybackRate] = オーディオプレイヤー!.rate
MPNowPlayingInfoCenter.default().nowPlayingInfo = nowPlayingInfo
}
func setMPRemoteCommandCenter() {
let commandCenter = MPRemoteCommandCenter.shared()
commandCenter.playCommand.addTarget { [unowned self] event in // 👈 3
print("play")
オーディオプレイヤー!.play()
return .success
}
commandCenter.pauseCommand.addTarget { [unowned self] event in // 👈 4
print("pause")
オーディオプレイヤー!.pause()
return .success
}
commandCenter.nextTrackCommand.addTarget{ [unowned self] event in // 👈 5
print("next")
// ここに次の曲に進む処理を記述
return .success
}
commandCenter.previousTrackCommand.addTarget{ [unowned self] event in // 👈 6
print("previous")
// ここに前の曲に戻る処理を記述
return .success
}
commandCenter.changePlaybackPositionCommand.addTarget { (event) -> MPRemoteCommandHandlerStatus in // 👈 7
print("seek")
if let changePlaybackPositionCommandEvent = event as? MPChangePlaybackPositionCommandEvent {
let positionTime = changePlaybackPositionCommandEvent.positionTime
self.オーディオプレイヤー!.currentTime = positionTime
MPNowPlayingInfoCenter.default().nowPlayingInfo![MPNowPlayingInfoPropertyElapsedPlaybackTime] = positionTime
}
return .success
}
}
}
公式 : MPRemoteCommandcenter、MPRemoteCommand
まとめ
SwiftUIでコントロールセンターに音声ファイルのタイトル / アルバム名を表示し、再生 / 停止 / スキップ / シークを実行できるようにする方法を説明した。
コメント